CultureInfo class provides culture-specific information in .NET applications. This information includes language, sublanguage, country or region, day names, month names, calendar etc. It also provides culture specific conversions of numbers, currencies, dates or strings. In the following tutorial, I will show you how you can retrieve this information from different cultures available in .NET Framework.
To use CultureInfo class you need to import System.Globalization namespace which contains many classes used in the following code such as RegionInfo, DateTimeFormatInfo or NumberFormatInfo.
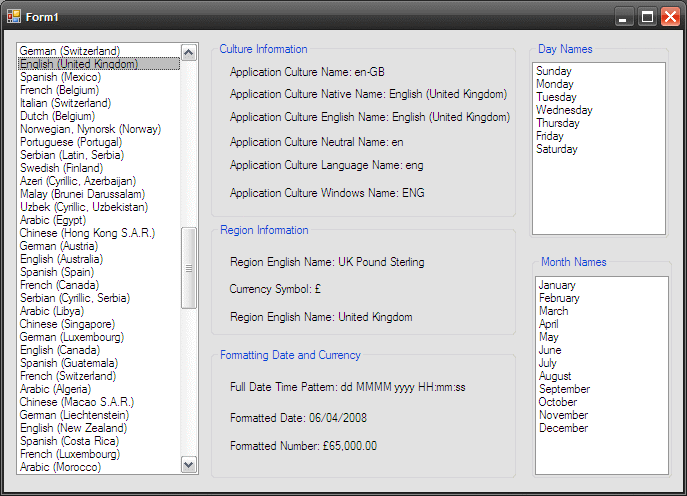
Following code is loading all specific cultures available in .NET Framework in the listbox.
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
' Load All Specific Cultures
Dim allCultures As CultureInfo() = CultureInfo.GetCultures(CultureTypes.SpecificCultures)
For Each culture As CultureInfo In allCultures
listBox1.Items.Add(culture.EnglishName)
Next
End Sub
When user select any culture from the listbox following code retrieve that culture, set current application and user interface culture and then display culture specific information on different labels.
Private Sub listBox1_SelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
Try
Dim allCultures As CultureInfo() = CultureInfo.GetCultures(CultureTypes.SpecificCultures)
Dim index As Integer = listBox1.SelectedIndex
Dim c As CultureInfo = DirectCast(allCultures.GetValue(index), CultureInfo)
' Set Current Application and User Interface Culture
System.Threading.Thread.CurrentThread.CurrentCulture = c
System.Threading.Thread.CurrentThread.CurrentUICulture = c
label1.Text = "Application Culture Name: " + c.Name
label2.Text = "Application Culture Native Name: " + c.NativeName
label3.Text = "Application Culture English Name: " + c.EnglishName
label4.Text = "Application Culture Neutral Name: " + c.TwoLetterISOLanguageName
label5.Text = "Application Culture Language Name: " + c.ThreeLetterISOLanguageName
label6.Text = "Application Culture Windows Name: " + c.ThreeLetterWindowsLanguageName
' RegionInfo Object
Dim r As New RegionInfo(c.Name)
label7.Text = "Region English Name: " + r.CurrencyEnglishName
label8.Text = "Currency Symbol: " + r.CurrencySymbol
label9.Text = "Region English Name: " + r.EnglishName
' DateTimeFormatInfo Object is used
' to get DayNames and MonthNames
Dim days As String() = c.DateTimeFormat.DayNames
listBox2.Items.Clear()
For Each day As String In days
listBox2.Items.Add(day)
Next
Dim months As String() = c.DateTimeFormat.MonthNames
listBox3.Items.Clear()
For Each month As String In months
listBox3.Items.Add(month)
Next
' Formatting Currency and Numbers
label10.Text = "Full Date Time Pattern: " + c.DateTimeFormat.FullDateTimePattern
label11.Text = "Formatted Date: " + DateTime.Now.ToString("d", c.DateTimeFormat)
label12.Text = "Formatted Number: " + 65000.ToString("C", c.NumberFormat)
Catch ex As Exception
End Try
End Sub
Great and useful
thanks
Great and useful
thanks
Great!!!
its very nice, i like your work. thank you for sharing.
Great!!!
its very nice, i like your work. thank you for sharing.
I realized there was no handles mybase.load on the load sub and no handles listbox1.selectedIndexChanged.
Added those codes and it worked gracefully.
Thanks for the tutorials. It was great.
Hi…
I’m sorry. i tried your code but was unsuccessful.
When i run the application, listbox1 was not populated. I imported system.globalization namespace, copied and pasted the form load sub, and listbox sub.
Where did i go wrong?
I realized there was no handles mybase.load on the load sub and no handles listbox1.selectedIndexChanged.
Added those codes and it worked gracefully.
Thanks for the tutorials. It was great.
Hi…
I’m sorry. i tried your code but was unsuccessful.
When i run the application, listbox1 was not populated. I imported system.globalization namespace, copied and pasted the form load sub, and listbox sub.
Where did i go wrong?
Best
This Coding Is Excellent , Great Job Man.
This Coding Is Excellent , Great Job Man.
Thank you.
Thank you.
use
Dim r As New RegionInfo(c.LCID)
instead of
Dim r As New RegionInfo(c.Name)
use
Dim r As New RegionInfo(c.LCID)
instead of
Dim r As New RegionInfo(c.Name)
too good. hats off 2 u