CultureInfo class provides culture-specific information in .NET applications. This information includes language, sublanguage, country or region, day names, month names, calendar etc. It also provides culture specific conversions of numbers, currencies, dates or strings. In the following tutorial, I will show you how you can retrieve this information from different cultures available in .NET Framework.
To use CultureInfo class you need to import System.Globalization namespace which contains many classes used in the following code such as RegionInfo, DateTimeFormatInfo or NumberFormatInfo.
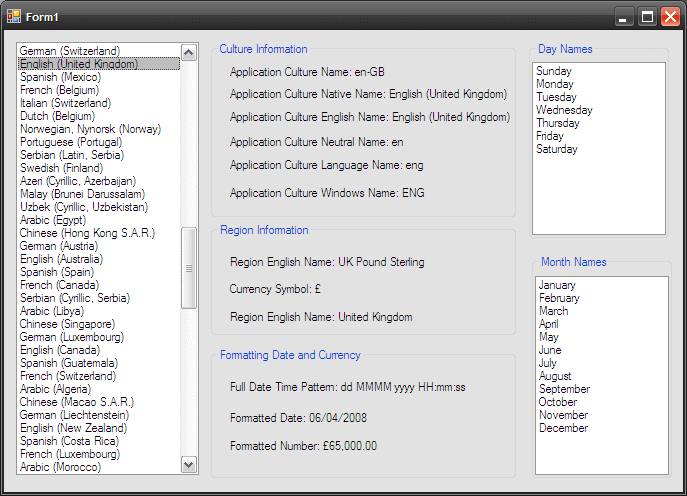
Following code is loading all specific cultures available in .NET Framework in the listbox.
private void Form1_Load(object sender, EventArgs e)
{
// Load All Specific Cultures
CultureInfo[] allCultures = CultureInfo.GetCultures(CultureTypes.SpecificCultures);
foreach (CultureInfo culture in allCultures)
{
listBox1.Items.Add(culture.EnglishName );
}
}
When user select any culture from the listbox following code retrieve that culture, set current application and user interface culture and then display culture specific information on different labels.
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
try
{
CultureInfo[] allCultures = CultureInfo.GetCultures(CultureTypes.SpecificCultures);
int index = listBox1.SelectedIndex;
CultureInfo c = (CultureInfo)allCultures.GetValue(index);
// Set Current Application and User Interface Culture
System.Threading.Thread.CurrentThread.CurrentCulture = c;
System.Threading.Thread.CurrentThread.CurrentUICulture = c;
label1.Text = "Application Culture Name: " + c.Name;
label2.Text = "Application Culture Native Name: " + c.NativeName;
label3.Text = "Application Culture English Name: " + c.EnglishName;
label4.Text = "Application Culture Neutral Name: " + c.TwoLetterISOLanguageName;
label5.Text = "Application Culture Language Name: " + c.ThreeLetterISOLanguageName;
label6.Text = "Application Culture Windows Name: " + c.ThreeLetterWindowsLanguageName;
// RegionInfo Object
RegionInfo r = new RegionInfo(c.Name);
label7.Text = "Region English Name: " + r.CurrencyEnglishName;
label8.Text = "Currency Symbol: " + r.CurrencySymbol;
label9.Text = "Region English Name: " + r.EnglishName;
// DateTimeFormatInfo Object is used
// to get DayNames and MonthNames
string[] days = c.DateTimeFormat.DayNames;
listBox2.Items.Clear();
foreach (string day in days)
{
listBox2.Items.Add(day);
}
string[] months = c.DateTimeFormat.MonthNames;
listBox3.Items.Clear();
foreach (string month in months)
{
listBox3.Items.Add(month);
}
// Formatting Currency and Numbers
label10.Text = "Full Date Time Pattern: " + c.DateTimeFormat.FullDateTimePattern;
label11.Text = "Formatted Date: " + DateTime.Now.ToString("d", c.DateTimeFormat);
label12.Text = "Formatted Number: " + 65000.ToString("C", c.NumberFormat);
}
catch (Exception ex)
{ }
}
Hi, i like this one but if i want to translate the Text box item that i have entered , then am not getting . please help me out.
Hi there m8, firstly thanks for this little app it has helped me alot to better understand the CultureInfo class and how to use it. I have one question though. What does the the two System.Threading.Thread.CurrentThread.CurrentCulture lines of code do? Thanks.
This is really nice article to understand the Culture.
I like your tutorial very much!
Is it possible to get a copy of your project, then i dont have to do it from scratch – its exactly that kind of thing i have been looking for!
Best Regards
Bjarne Pedersen
thx
Very Nice Article. It helped me a lot
Thanks
thx. for ur code………. code is just copied from microsoft book…..am looking for indepth code which will display all UI in selected language
its v good tutorial!!!
Very good tutorial
Thank you very much 🙂
Nice Article…
Thanx 🙂
thank
Thanks for giveCultureInfo class in C# to implement Globalization tutorial,it is helpful for learning dotnet 2.0.
Thanks
Santosh Kumar
http://www.operativesystems…
Thanks for giveCultureInfo class in C# to implement Globalization tutorial